Redux
1.Actions & Action Creators
//action type
const ADD_TODO = 'ADD_TODO';
//action creator, semantic methods that create actions
//collected together in a module to become an API
function addTodoAction(title, hour) {
//action, an object with a type property and new data, like events
return {type: ADD_TODO, title, hour}
}
2.Reducers
//a function that accepts an accumulation and a value and returns a new accumulation.
function todoReducers(state = [], action) {
switch (action.type) {
case ADD_TODO:
//always return a new state, never mutate old state
return [
{
id: Utils.GUID(),
title: action.title,
endTime: getEndTime(action.hour),
completed: false
},
...state
]
default:
//return default state
return state
}
}
3.Store
import { createStore } from 'redux';
//1. define store
let store = createStore(todoReducers);
class App extends Component {
constructor(props){
super(props);
this.state = {todos: []};
}
componentDidMount(){
//2. subscribe store
this.unsubscribeStore = store.subscribe(() =>{
//3. getState
this.setState({todos: store.getState()});
});
}
componentWillUnmount(){
//5. unsubscribe store
this.unsubscribeStore();
}
renderTodoList = ()=>{
//reder todo list
return this.state.todos.map( (todo)=> {
return <Text key={todo.id}>Todo: {todo.title}</Text>
});
}
handleAddTodo = ()=>{
//4. dispatching actions
store.dispatch( addTodoAction('Create a new todo', 8) );
}
render() {
return (
<View>
<TouchableHighlight onPress={this.handleAddTodo}>
<Text>Add Todo</Text>
</TouchableHighlight>
<ScrollView>{this.renderTodoList()}</ScrollView>
</View>
);
}
}
4.Data flow
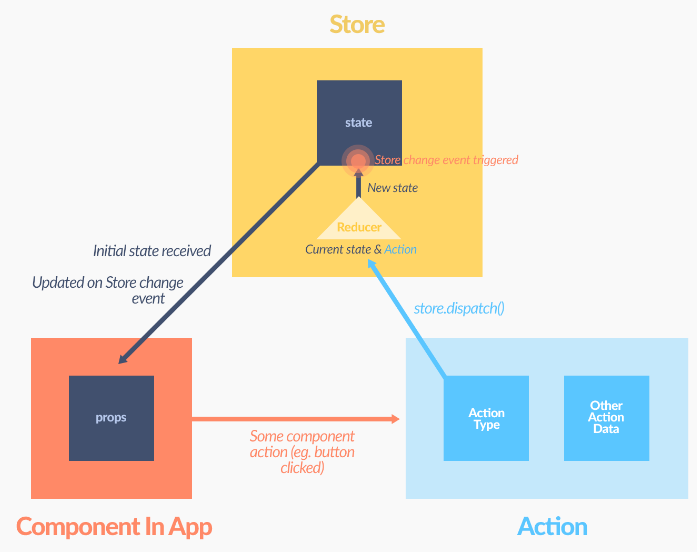
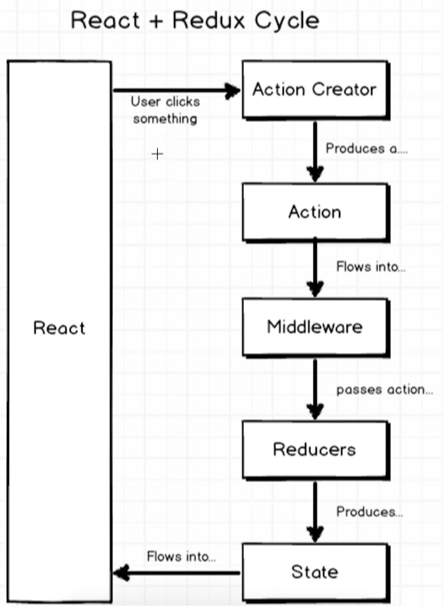
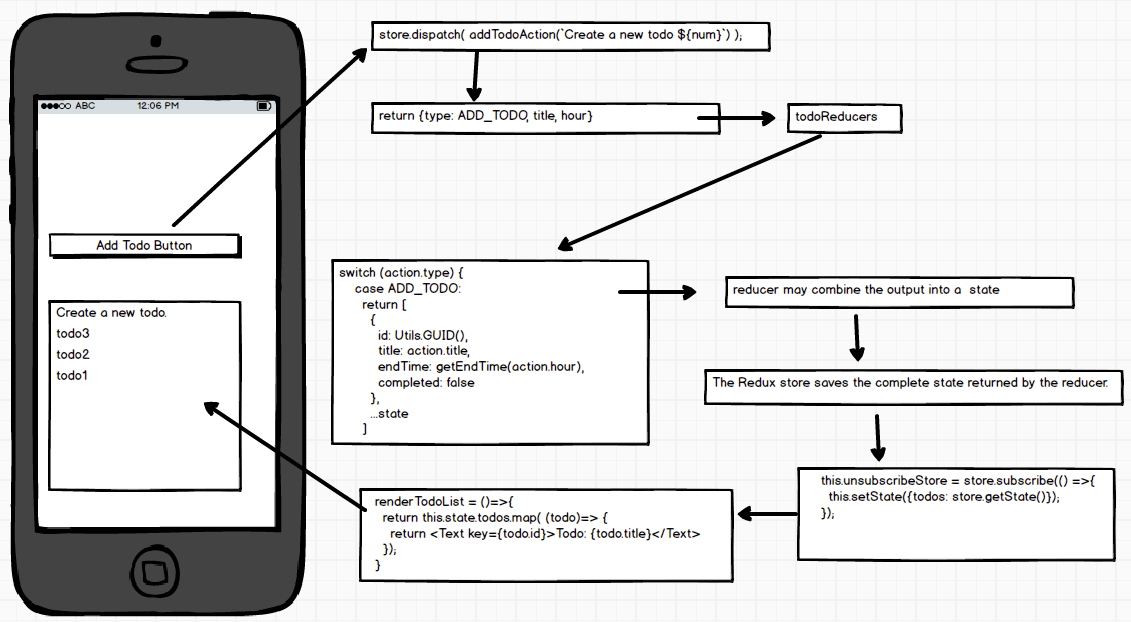